Martin Fowler once said “Anyone can write a code that a computer can understand. Good programmers write code that humans can understand.”
Clean code is an essential component of software development.
Writing clean code is exactly like a sales pitch. When you use words full of technical jargon, you end up losing your target audience. The same is true with coding as well. Writing clean code enhances the readability, maintainability, and understandability of the software.
What is clean code?
Robert C. Martin in his book “Clean Code: A Handbook of Agile Software Craftsmanship“ defined clean code as:
“A code that has been taken care of. Someone has taken the time to keep it simple and orderly. They have laid appropriate attention to details. They have cared.”
Clean code is clear, understandable, and maintainable. It is well-organized, properly documented, and follows standard conventions. The purpose behind clean code is to create software that is not just functional but readable and efficient throughout its lifecycle. Since the audience isn’t a computer but rather a real live audience.
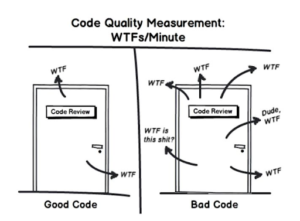
Why is clean code important?
Clean code is the foundation of sustainable software development. Below are a few reasons why clean code is important:
Reduce technical debt
Technical debt can slow down the development process in the long run. Having clean code ensures that future modifications will be smoother as well as less costly process.
Increase code readability and maintainability
Clean code means that the developers are prioritizing clarity. When it is easier to read, understand, and modify code, it leads to faster software development.
Enhance collaboration
Good code means that the code is accessible to all team members and follows coding standards. This helps in improved communication and collaboration among them.
Debugging and issue resolution
Clean code is designed with clarity and simplicity. Hence, making it easier to locate and understand specific sections of the codebase. This further helps in identifying and resolving issues in the early stages.
Ease of testing
Clean code facilitates unit testing, integrated testing, and other forms of automated testing. Hence, leading to increased reliability and maintainability of the software.
Clean code principles and best practices
Below are some established clean code principles that most developers find useful.
KISS rule
Apply the KISS (Keep it simple, stupid) rule. It is one of the oldest principles of clean code. This means that don’t make the code unnecessarily complex. Make it as simple as possible. So that it takes less time to write, has less chance of bugs, easier to understand and modify.
Curly’s law
This law states that the entity (class, function, or variable) must have a single, defined goal. It should only do one thing in one circumstance.
DRY rule
DRY (Don’t repeat yourself) is closely related to the KISS rule and Curly’s law. It states to avoid unnecessary repetition or duplication of code. Not following this can make the code prone to bugs and make the code change difficult.
YAGNI rule
YAGNI (You aren’t gonna need it) rule is an extreme programming practice that states that the developers shouldn’t add functionality unless deemed necessary. It should be used in conjunction with continuous refactoring, unit testing, and integration.
Fail fast
It means that the code should fail as early as possible. This is because issues can be quickly identified and resolved which further limits the number of bugs that make it into production.
Boy scout rule
This rule by Uncle Bob states that always leave the code cleaner than you found it. It means that software developers must incrementally improve parts of the codebase they interact with, no matter how minute the enhancement might be.
SOLID principles
Apply the SOLID principles. This refers to:
S: Single Responsibility Principle which means that the classes must only have a single responsibility.
O: The open-closed Principle states that the piece of software should be open for extension but closed for modification.
L: The Liskov Substitution Principle means that subclasses should be able to substitute their base class without getting incorrect results.
I: The Interface Segregation Principle states that interfaces should be specific to clients instead of being generic for all clients.
D: The dependency Inversion Principle means that classes should depend on abstractions (interfaces) rather than concrete implementations.
A few of the best practices include:
Use descriptive and meaningful names
Choose descriptive and clear names for variables, functions, classes, and other identifiers. They should be easy to remember and according to the context that conveys the purpose and behavior to make the code understandable.
Follow established code-writing standards
Most programming languages have community-accepted coding standards and style guides. Some of them include Google Java style and PEP 8 for Python and Javascript. Organizations must also have internal coding rules and standards that provide guidelines for consistent formal, naming conventions and overall code organization.
Avoid writing unnecessary comments
Comments help explain the code. However, the codebase changes continuously so the comment can become old or obsolete soon. This can create confusion and distraction among software developers. Make sure to keep the comments updated. Also, avoid writing poorly written or redundant comments as it may increase the cognitive load of software engineering teams.
Avoid magic numbers
Magic numbers are hard-coded numbers in code. They are considered to be a bad practice since they can cause ambiguity and confusion among developers. Instead of directly using them, create symbolic constants for hard-coded values. It makes it easy to change the value at a later stage and improves the readability and maintainability of the code.
Refactor continuously
Ensure that you regularly refactor to enhance the structure and readability of the code. It also helps in improving its flexibility and maintaining code that is overly complex, poorly structured, or duplicated.
You can apply refactoring techniques such as extracting methods, renaming variables, and consolidating duplicate code to keep the codebase cleaner.
Version control
Version control systems such as GIT, SVN, and Mercurial help track changes to your code and pull back to previous versions, if necessary. Before refactoring, ensure that the code is under version control to safely experiment with changes. Moreover, it helps understand the evolution of the project and maintains the integrity of the codebase by enforcing a structured workflow.
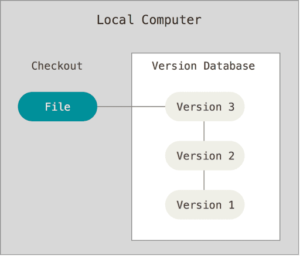
Testing
Software developers can write unit tests to verify the code’s correctness as well-tested code is reliable and easier to refactor. Test-driven development helps in writing cleaner code as it considers edge cases and provides immediate feedback on code changes.
Code reviews
Code reviewing continuously helps in ensuring code quality by identifying potential issues, catching bugs, and enforcing coding standards. It also facilitates collaboration between software developers to see each other’s strengths and review mistakes together.
Typo - An automated code review tool
Typo’s automated code review tool not only enables developers to catch issues related to code maintainability, readability, and potential bugs but also can detect code smells. It identifies issues in the code and auto-fixes them before you merge to master. This means less time reviewing and more time for important tasks. It keeps the code error-free, making the whole process faster and smoother.
Key features:
- Supports top 10+ languages including JS, Python, Ruby
- Understands the context of the code and fixes issues accurately
- Optimizes code efficiently
- Standardizes code and reduces the risk of a security breach
- Provides automated debugging with detailed explanations
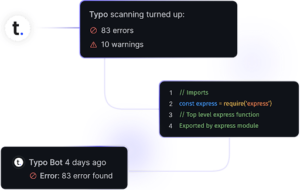
Conclusion
Writing clean code isn’t just a crucial skill for developers. It is an important way to sustain software development projects.
By following the above-mentioned principles and best practices, you can develop a habit of writing clean code. It will take time but it will be worth it in the end.
Hope this was helpful. All the best!